How to Get the Hang of JavaScript Functions
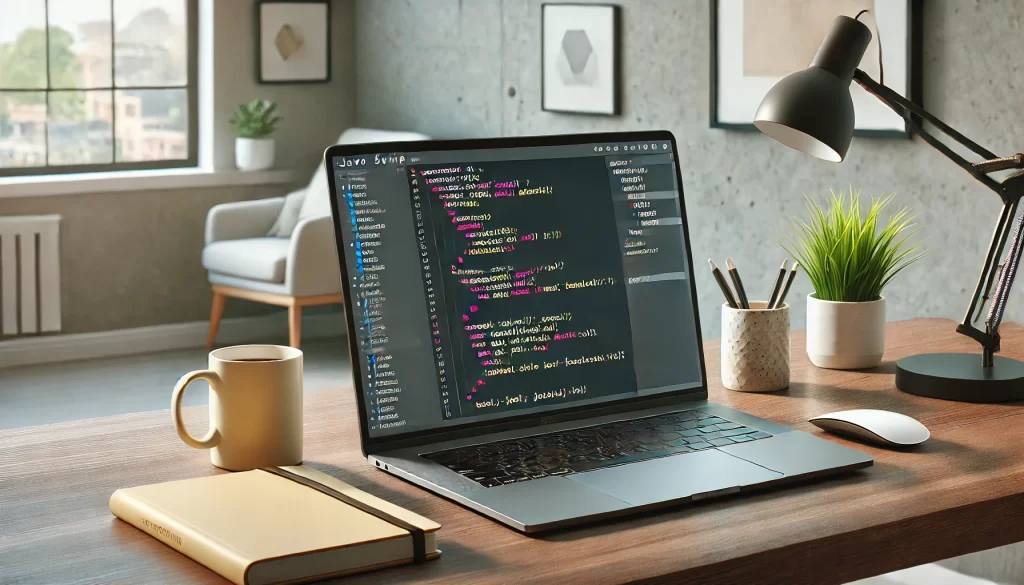
Why Use Functions in JavaScript?
Functions are the backbone of JavaScript programming. You’ll be using them everywhere—like spices in a good chili. They bring order to chaos by bundling reusable blocks of code into neat little packages.
Without them, you’d be stuck copying and pasting lines all over the place, and every time you needed to tweak something, you’d have to hunt down every instance of that code. It’s like trying to repair a leaky roof by patching one shingle at a time—you’re going to miss a spot.
Functions allow you to encapsulate chunks of logic so you can call them whenever and wherever you want. They ensure that if you need to update or change something, you only have to do it once. Pretty slick, huh?
Creating and Calling Functions
So, how do you create one of these bad boys? It’s easier than baking a pie. You start with the keyword function
, give it a name (kind of like naming a pet), and then wrap your logic inside a pair of curly braces. For example:
function sayHi() {
console.log("Hi!");
}
This code doesn’t actually *do* anything yet, but you’ve defined a function. Now, to make it run, you call it by name, like this:
sayHi();
Bam! Every time you call sayHi()
, your message “Hi!” will pop out like a jack-in-the-box.
Function Arguments: Making Functions Flexible
Now, what if you want your function to be a little more flexible—like making it say “Hi” sometimes and “Hello” other times? You can pass arguments into the function. This is like giving your function some ingredients to work with. Here’s how you’d do it:
function greet(greeting) {
console.log(greeting);
}
greet("Hello!");
greet("Hi there!");
By passing in different arguments, you can customize what your function outputs. It’s like ordering pizza with different toppings—same pizza, different flavors.
Returning Values from Functions
Okay, so your function can now print stuff, but what if you want it to give something back—like a result you can use later? That’s where the return
statement comes in. Here’s an example:
function add(a, b) {
return a + b;
}
let sum = add(5, 10);
console.log(sum); // Outputs 15
See how that works? The function takes two numbers, adds them, and then returns the result, which you can use wherever you like. The return
statement is like a vending machine—it gives you back something based on what you put in.
Best Practices for Using Functions
Before I let you loose with all this newfound power, let’s talk about some best practices. First, give your functions clear, descriptive names. If you call a function doThing()
, future-you will have no idea what it was supposed to do. It’s like labeling everything in your garage as “stuff.” Be specific.
Second, keep your functions focused. Each one should do one thing and do it well—don’t make them multitask. A function that tries to do too much is like a Swiss Army knife with too many tools—you’ll cut yourself.
Have some questions? Click here to get FREE personalized answers.
This article was created with the aid of AI tools.
Leave a Reply
You must be logged in to post a comment.